Now that I have node components working in SpriteKit, it’s time to add something more dynamic than tapping to add a node that then doesn’t do anything.
Let’s get…physical.
(sorry.)
Creating a Physics Component
I’ll pick up where we left off with the generic node component and use the same Playground that’s available on Github.
In keeping with the idea that components should describe attributes and abilities of entities, the presence of a physics component will define whether or not an entity is even part of the physics engine.
We’ll start with this PhysicsComponent
:
class PhysicsComponent : GKComponent { var body : SKPhysicsBody? // 1. init( body : SKPhysicsBody ) { self.body = body super.init() } required init?(coder aDecoder: NSCoder) { fatalError("Not implemented") } // 2. override func didAddToEntity() { self.entity?.component(ofType: NodeComponent.self)?.node.physicsBody = self.body } // 3. override func willRemoveFromEntity() { if let hasNode = self.entity?.component(ofType: NodeComponent.self)?.node { hasNode.physicsBody = nil // 4. self.entity?.component(ofType: PositionComponent.self)?.currentPosition = hasNode.position } self.body = nil } }
- The component will have no idea what kind of node it will be attached to, so the size and shape of the body will need to be decided by whatever creates the entity.
- When this component gets added to an entity, it checks to see if there’s a node component (which should be the root node of the entity), and applies the physics body to it.
- If the component is ever removed, this should be taken as a sign that we don’t want the entity to participate in the physics system any more (whether or not the node itself was removed from the scene is irrelevant).
- Our
PositionComponent
is out of sync with the node’s actual position because it’s been the physics system moving it. We should update our position component to be the same as the node’s current position so that it stays wherever it is and doesn’t snap back to where it started.
Next, we’ll add it to some entities.
In the addNode(to:)
function of the GameScene
class, before the line entities.append(entity)
, we’ll add:
let body = SKPhysicsBody(rectangleOf: nodeComponent.node.calculateAccumulatedFrame().size) let physics = PhysicsComponent(body: body) entity.addComponent(physics)
This will get the physics working.
Kind of:
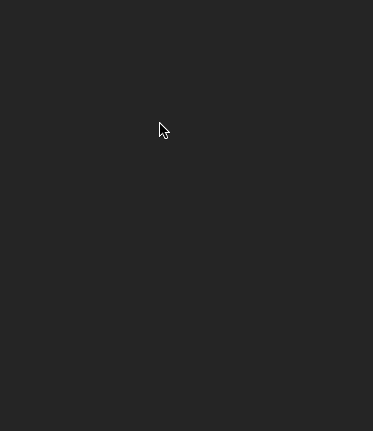
Hmm. Gravity is usually a lot stronger than that.
And why is the square rotating randomly when I add the circle?
Don’t Cross The Streams
Let’s go back to the famous SpriteKit update loop:
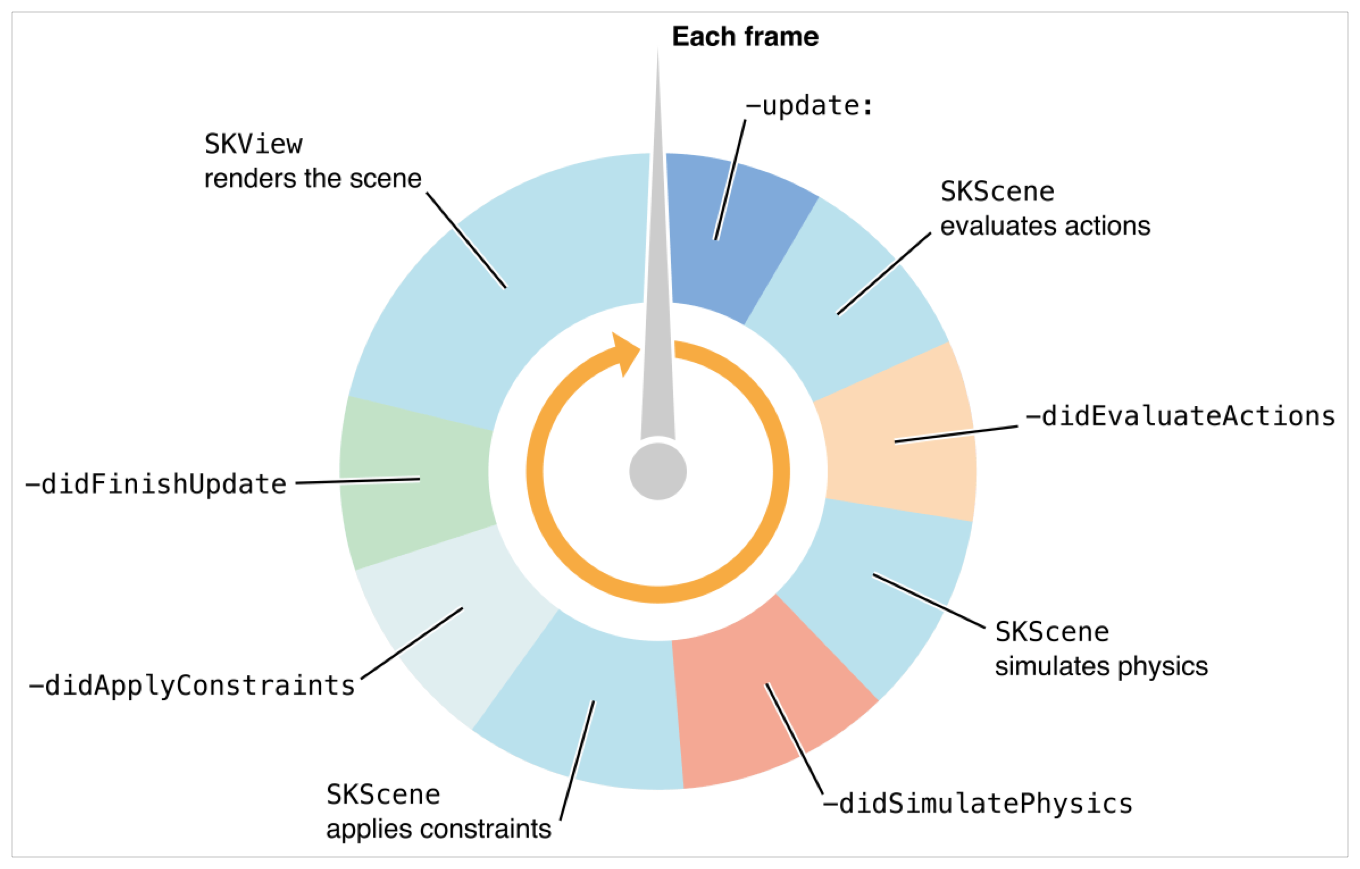
I have a position component that is being run in that first update(_:)
method every frame. The position component is applying its current position (which doesn’t change) to the node at the start of every frame.
The SKScene
simulates the physics, and updates the position of the node based on the results of that simulation.
Finally, SKView
renders the scene using the node’s position, last updated by the physics engine.
At the start of the next frame, the position component puts the node right back where it started, and we go through the whole process again.
Shouldn’t The Node Stay In The Same Place, Then?
Let’s have a look. At the bottom of the GameScene
class, we’ll add:
override func didSimulatePhysics() { guard let hasEntity = self.entities.first else { return } print("---PHYSICS APPLIED---") print( hasEntity.component(ofType: PositionComponent.self)?.currentPosition ?? "No position") print( hasEntity.component(ofType: NodeComponent.self)?.node.position ?? "No node") print( hasEntity.component(ofType: PhysicsComponent.self)?.body?.velocity ?? "No velocity") }
If I override the didSimulatePhysics()
method and then print out some information about the body, we can see what’s happening:
---PHYSICS APPLIED--- (198.50001525878906, 402.5000305175781) (198.50001525878906, 261.8658447265625) (0.0, -8440.6572265625) ---PHYSICS APPLIED--- (198.50001525878906, 402.5000305175781) (198.50001525878906, 261.6921691894531) (0.0, -8451.0751953125)
The physics engine is not just updating the position of the node, it’s updating its velocity as well and using that in its calculation for where it wants to position it.
As gravity is working its magic on the body, the y-velocity of our node is increasing every frame.
However, at the start of every frame, the position component puts the box back at its initial point. Then the physics component updates the node’s position again based on how far it would travel in the fraction of a second between frames given its (rapidly increasing) velocity. The frame renderer paints the box on screen at the location which is the result of this calculation.
If I update the NodeComponent
’s update(deltaTime:)
method (which, remember, was acting as my render system) to look like this:
override func update(deltaTime seconds: TimeInterval) { guard self.entity?.component(ofType: PhysicsComponent.self) == nil else { return } if let hasPos = self.entity?.component(ofType: PositionComponent.self)?.currentPosition { self.node.position = hasPos } }
And I make a quick update to the PositionComponent
to apply its initial position to the node when it’s added to an entity:
override func didAddToEntity() { self.entity?.component(ofType: NodeComponent.self)?.node.position = self.currentPosition }
Everything works like it should:
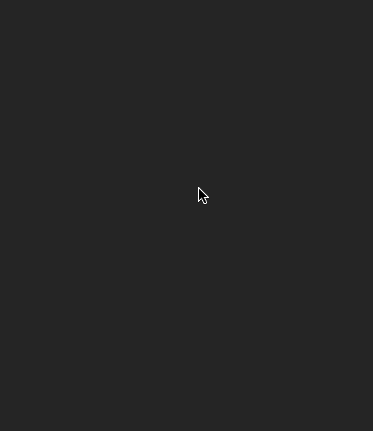
When we are working with SpriteKit, the golden rule is that we can either update the position of our nodes manually and not use physics or we relinquish control and let the physics engine do it. We can’t do both.
But What If I Want Control?
Then we need to work with the physics system. First, in the PhysicsComponent
, we’ll add a new property called position
:
var position : CGPoint?
It’s an optional CGPoint
because we want to use nil as an indicator that the manual interaction is over.
Then, underneath the closing brace of the the GameScene
class, we’ll add this new class:
class TouchyNode : SKNode { override func touchesBegan(_ touches: Set, with event: UIEvent?) { // 1. guard let t = touches.first, let scene = self.scene else { return } // 2. self.entity?.component(ofType: PhysicsComponent.self)?.position = t.location(in: scene) } override func touchesMoved(_ touches: Set , with event: UIEvent?) { guard let t = touches.first, let scene = self.scene else { return } // 3. if self.entity?.component(ofType: PhysicsComponent.self)?.position == nil { return } self.entity?.component(ofType: PhysicsComponent.self)?.position = t.location(in: scene) } override func touchesEnded(_ touches: Set , with event: UIEvent?) { // 4. self.entity?.component(ofType: PhysicsComponent.self)?.position = nil } }
- Because we’re dealing with a single small node, I’m only going to be interested in the first touch. Also, note that this first node in the tree has a reference to the scene its placed in. Useful.
- Convert the location of the touch (which will be in the node’s co-ordinate system) into the scene’s co-ordinate system and then apply it to the new Physics position property
- This method is the same as the
touchesBegan(_:with:)
method except for this bit: There may be a time where we want to remove control from the player (for example having a projectile knock the object we’re moving out of our “hand”). Another system could do this by setting the physics position property to nil. However, if the player doesn’t end the touch themselves,touchesEnded(_:with:)
doesn’t get called. We therefore need to manually check here to see if theposition
property is nil. If it is, the touch has been cancelled by another system and we can bail out early. - If the player does end the touch, then we set the position property to nil ourselves which indicates that full control should return to the physics engine.
Next, we want to actually use our new TouchyNode
. Replace the current NodeComponent
with:
class NodeComponent : GKComponent { let node = TouchyNode() override func didAddToEntity() { // 1. self.node.entity = self.entity // 2. self.node.isUserInteractionEnabled = true } override func willRemoveFromEntity() { self.node.entity = nil } }
- We’ll want a reference to the entity in the node, as it is the node that will be responding to touches (not the entity).
- We also have to manually enable
isUserInteractionEnabled
on the node as it’s off by default (meaning the touches within the node will be ignored).
Then replace the NodeComponent
extension (for the second time this post) with:
extension NodeComponent { override func update(deltaTime seconds: TimeInterval) { // 1. if let physicsComponent = self.entity?.component(ofType: PhysicsComponent.self) { // 2. guard let hasPhysicsPosition = physicsComponent.position else { return } // 3. let distance = CGVector(dx: hasPhysicsPosition.x - self.node.position.x, dy: hasPhysicsPosition.y - self.node.position.y) let velocity = CGVector(dx: distance.dx / CGFloat(seconds), dy: distance.dy / CGFloat(seconds)) // 4. physicsComponent.body?.velocity = velocity } else { if let hasPos = self.entity?.component(ofType: PositionComponent.self)?.currentPosition { self.node.position = hasPos } } } }
- If we have a physics component, we’re positioning using physics properties and not manually so we need to check for this first.
- If the physics position is nil, the engine is back in full control so we do nothing and let the engine work it out.
- Do some math: speed = distance / time. The distance is the difference between the touch position and the current node position, and the time is the time between frames (these lines are adapted from this Stack Overflow answer).
- Apply this newly calculated velocity to the physics body.
Now, when the physics engine gets hold of the node (remember the physics are simulated after we’ve done all this), the velocity will be set perfectly to move the node from where it currently is to where our finger is each and every frame.
It also means our node works perfectly with any existing physics bodies, and it will maintain momentum if we let go:
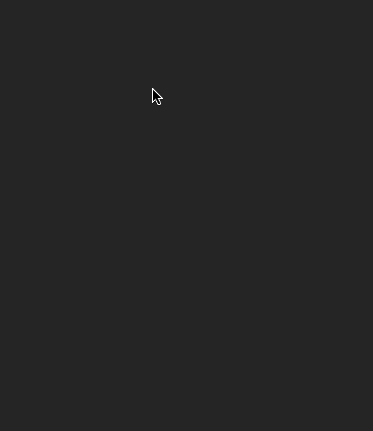
Now we have a working physics system using ECS!
But, really, labels shouldn’t participate in the physics simulation! They’re labels!
Let’s replace:
let body = SKPhysicsBody(rectangleOf: nodeComponent.node.calculateAccumulatedFrame().size) let physics = PhysicsComponent(body: body) entity.addComponent(physics)
With:
if type != .label { let body = SKPhysicsBody(rectangleOf: nodeComponent.node.calculateAccumulatedFrame().size) let physics = PhysicsComponent(body: body) entity.addComponent(physics) }
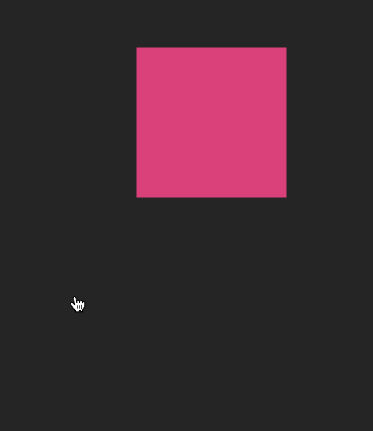
Better! The physics component’s presence defines whether or not the entity it belongs to should take part in a physics simulation. Better yet, they can also be added or removed dynamically to switch the physics for an entity on or off.
These components are getting interesting! Let’s see what else they can do…