My second adventure game prototype is a traditional 2D adventure engine designed to explore whether the following are feasible:
- Developing it as a CocoaPod for reuse over a number of games
- Using a standard JSON format that would describe room parameters (e.g. walkable areas, object locations, NPC locations, environmental triggers, etc.)
- Using a separate JSON format to describe dialogue trees, with support for triggers, branching dialogue, and basic conditionals
- Recreating something like this in C# for use in Unity
Developing it as a CocoaPod
While it’s not impossible, SpriteKit doesn’t play super well in a framework. A lot of the fileNamed
methods assume that you’re in the main bundle, and there are no additional bundle parameters like there are for, e.g., UIImage
.
This means that using the SpriteKit Scene Editor is basically a non-starter—everything will have to be handled in code. No GUI shortcuts for me!
Everything else worked as well as I would have hoped, although not being in the main bundle did trip me up a few times.
I was able to create the framework as a Cocoapod then use it in an example application in a separate folder. Using the path
parameter in the Podfile
is extremely helpful for developing Cocoapods:
pod 'AdventurePod', :path => '../AdventurePod/AdventurePod'
All of the files within the Cocoapods project are editable and any changes made in the example application are reflected back in the framework.
Designing Room Parameters
I sketched out the room data files on paper, then created this example room in JSON:
{ "roomName" : "default", "background" : "background", "walkableAreas" : [[0, 0], [1500,200]], "playerEntry" : [100,80], "characters" : [{ "position" : [1000, 120], "name" : "Sinister Guy", "sprite" : "sinisterGuy", "dialogueFile" : "sinister" }] }
I wrote a struct in Swift that recreated this format and used the wonderful new Codable
protocols to decode the room JSON into these structs. It was incredibly straightforward, especially now that CGPoints
and CGRects
support Codable
.
Right now, the `walkableAreas` object is just a rectangle, but in future it would be an array of points representing an arbitrary polygon. There are also no exits defined.
I can already imagine how I might design a reusable SKScene subclass that would read these structs and set up the rooms. I would be able to transition between these rooms simply by passing a new instance of the class the name of the next JSON file, which would be taken from the name of the exit that the player was using.
Then there is the ability to do on-device editing of a scene, which opens up some interesting iterative possibilities during development.
Dialogue
I have been working on a JSON-based dialogue editor for a while now and it already supports branching dialogue, triggers, basic conditionals, and multiple options.
The simplest solution would just to re-use the parser from this editor and customise it to this engine. However—although there’s no reason this wouldn’t work—it would take too much time to do this for the prototype, so I settled on hardcoding a basic script instead.
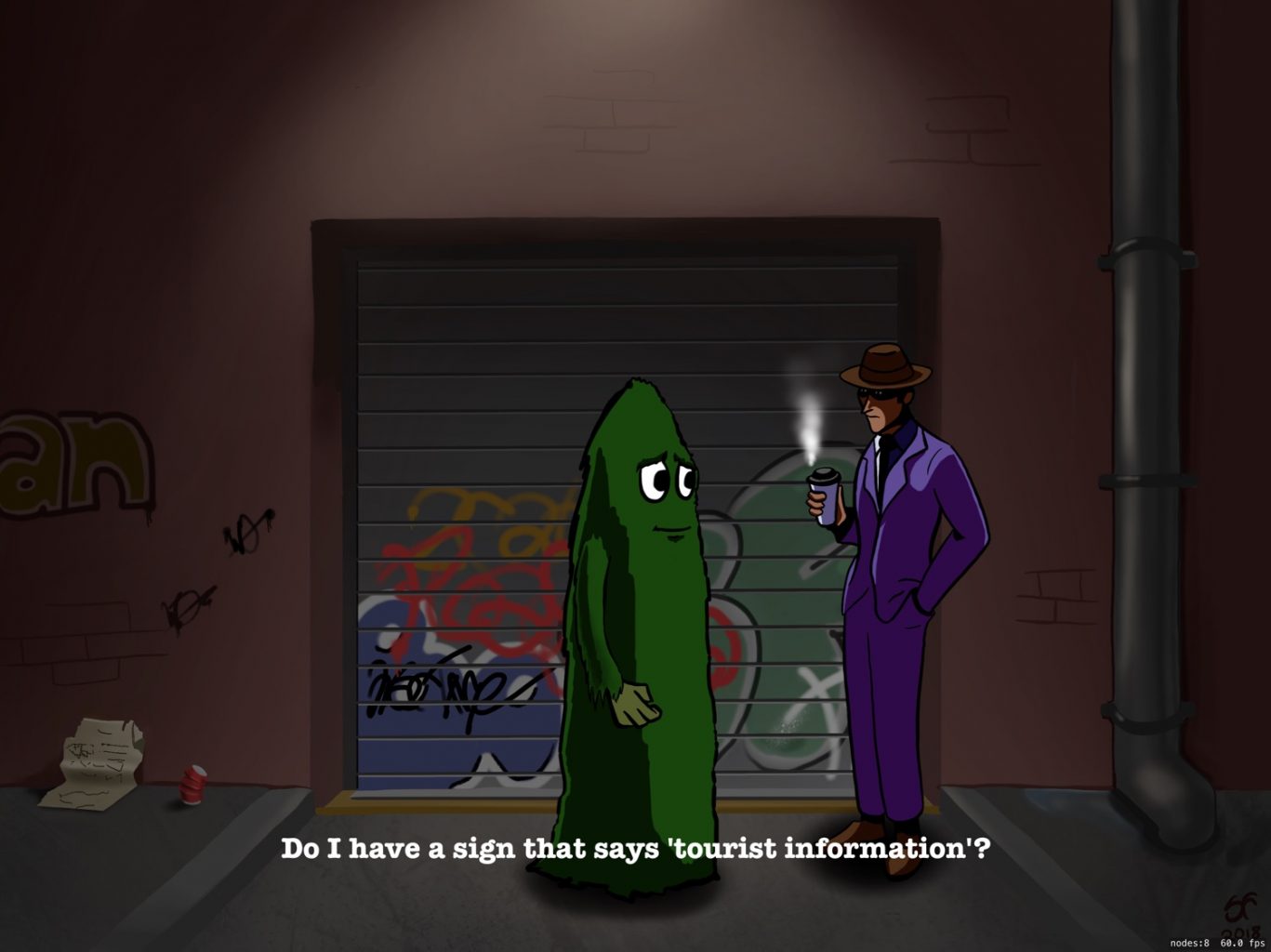
Development in C#
As there’s already a “no coding required” adventure game engine available in the Unity Asset Store, I’m going to go ahead and say that, yes, it’s feasible.
I wasn’t aware of this before I started but now that I know it exists, the next obvious question is why wouldn’t I just use this (other than I didn’t write it)?
Why indeed. This is something I’m going to be thinking about for a while…
Limitations and Challenges
This very basic sketch came together surprisingly quickly and I’m pleased that the fundamental idea seems solid. Of course, there are challenges:
- I purposely did not include any animation support. While not impossible, I have a feeling that this might complicate the JSON formats quickly.
There are also a couple of questions around device support:
- Because I can’t use any of the
fileNamed:
convenience methods, I believe it’s going to be up to the engine to determine the device scale and load the correct images rather than the system handling it automatically - Scaling backgrounds and playable areas to different device sizes—whether to require different assets for iPads or to use SpriteKit’s scaling and just handle the inevitable clipping that would come from reusing the same assets across devices
Having built both my prototypes and seeing the strengths of each, I now have a difficult decision to make: what do I want to spend the rest of the year working on? AR and 3D modelling or a 2D engine and some traditional drawing?
I think I’m going to put this decision off for a little while longer. Whichever I choose, they’re going to need a lot of dialogue, and I have some ideas for my dialogue editor that I’d love to implement first…